Introduction
Are you a software developer whose team has suffered from one of the following struggles?
- Is your documentation constantly out of date?
- Your documentation is scattered throughout multiple places and tools like Word, PDFs, wikis, Jira, etc?
- Writers are in a constant battle with you to sync with each other?
- There are new components and features, but you can't integrate them into your documentation?
“Docs as Code” may be the solution you need. This methodology is a modern approach that treats documentation like software code. Teams can create living, up-to-date, and maintainable documentation by integrating documentation into development workflows. This post will explore how this philosophy can improve software quality, the tools that make it possible, and how to adopt it successfully.
The old days
Historically, documentation has been one of those aspects of software development that produces more headaches. Some of the challenges developers or writers encounter when writing technical documentation are:
- Writing docs in different platforms and tools like Word, PDFs, wikis, or SaaS, separately from the source code.
- Disconnection between the development team and technical authors.
- Bottlenecks in reviews and updates due to manual processes.
- Struggles with version mismatches (e.g., docs for v1.0 while the product is at v2.0).
- Lack of version history.
This led to outdated docs, collaboration gaps, and friction in maintaining accuracy, ultimately affecting the quality and velocity of software development. Let’s see how the “Docs as Code” methodology can improve this.
Other traditional solutions have been CMS-based documentation platforms, these solutions lock your content into proprietary systems, lack flexibility, and usually, the performance is poor.
What Is Docs as Code?
Docs as Code (also referred to as DaC) treats documentation like software to make it more maintainable, scalable, and developer-friendly. It’s composed of the following practices/principles:
- Written in lightweight markup (e.g., Markdown, AsciiDoc).
- Stored in version control (e.g., Git) alongside code.
- Built, tested, and deployed using automation pipelines (CI/CD).
- Collaborated on code review processes (e.g., pull requests).
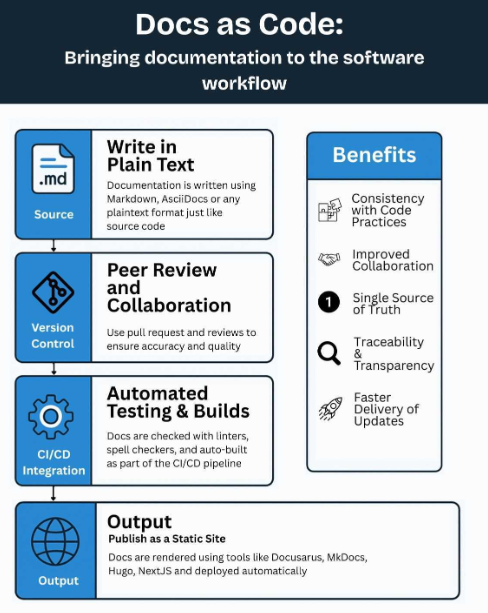
Why Docs as Code?
Docs as Code unlocks a lot of advantages that will improve your documentation, but also can improve the quality and speed of your software.
Improved Collaboration
Having your docs alongside code enables you to collaborate with different teams, developers, technical writers, and stakeholders using similar tools.
A lightweight format like Markdown makes it easy to modify by both humans and machines, which makes it the perfect format to automate documentation.
Version Control
Using version control tools like Git makes it easy to revert changes, work on the same file from different authors, resolve conflicts, and align docs with code releases.
Automation
You can use automatic tools we frequently use in software development to build, lint, and test your docs to catch early typos, broken links, etc.
Consistency
By leveraging re-usable templates and style guides, you can enforce consistency and uniformity across your docs.
Faster Feedback
The review process is similar to code, you get early feedback in “pull requests,” reducing delays. Tools like GitHub and GitLab support complex features over this.
Scalability
Makes it easy to build and maintain docs in large and fast-evolving environments.
Let’s build a Project
Let’s create a project that demonstrates how powerful the Docs as Code approach can be. For this example, we are going to create a small JavaScript application and add documentation files using MDX.
MDX is a superset of Markdown that includes JSX components, which enables to interleave documentation with live components. We’ll see some nice features this syntax can add to our documentation in the following steps.
Step 1: Scaffolding the Project
We’ll start with a fresh Vite project:
npm create vite@latest dac -- --template react-ts
cd dac
npm install
npm run dev # This start the development server
Visit http://localhost:5173/
and see the sample page:
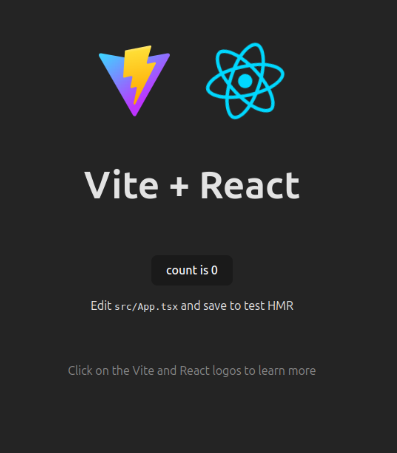
Step 2: Install Git and commit your changes
Having versioning control is one of the key features of DaC, so let’s use git to commit our files:
git init # Initialize the git repository
git add --all # Staging all files
git commit -m "Initial Commit" # Commit the changes with a description
This ensures that if we make any mistake, we can revert to a state where the application is working as expected.
Step 3: Adding an MDX file
Our project file structure looks like this:
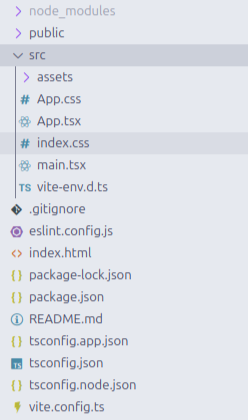
Let’s add a new file /src/docs/Readme.mdx:
# Hello, MDX!
This is normal **markdown** with _components_.
Nice! Now, how are we going to show this as part of our new application?
Step 4: Adding MDX Support
MDX requires a plugin to work with Vite. Let’s install the necessary package to make it work:
npm install @mdx-js/rollup
Then, update your vite.config.ts
to handle .mdx files:
import { defineConfig } from 'vite'
import react from '@vitejs/plugin-react'
import mdx from '@mdx-js/rollup';
// https://vite.dev/config/
export default defineConfig({
plugins: [react(), mdx()],
});
Step 5: Import MDX as a component
With the previous step, we can now import .mdx
files as if they were React components! Edit /src/App.tsx
file:
import './App.css'
import Readme from './docs/Readme.mdx'
function App() {
return (
<>
<div>
<Readme />
</div>
</>
)
}
export default App
You see the following screen:
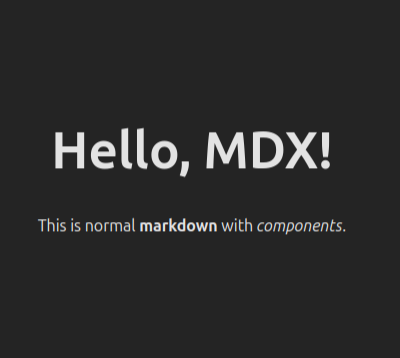
Great! Now we can import and render MDX files as if they were React components, but that’s not all! You can also import other components into MDX files and use JavaScript inside.
Let’s create a new React component at /src/components/Counter.tsx
import React, { useState } from 'react';
export const Counter: React.FC = () => {
const [count, setCount] = useState(0);
const increment = () => setCount(count + 1);
const decrement = () => setCount(count - 1);
return (
<div>
<h1>Counter: {count}</h1>
<button onClick={increment}>Increment</button>
<button onClick={decrement}>Decrement</button>
</div>
)
}
Then create a new .mdx
file at src/docs/Counter.mdx
import { Counter } from '../components/Counter';
# Counter Documentation
The `Counter` component is a simple React component that allows users to increment or decrement a counter value. It demonstrates the use of React's `useState` hook for managing state.
## Features
- Displays the current count.
- Provides buttons to increment and decrement the count.
## Usage
import { Counter } from './components/Counter';
function App() {
return (
<div>
<Counter />
</div>
);
}
## Example
<Counter />
If you replace the <Readme />
component in the App.tsx
files with the new <Counter />
mdx component, you will see something like:
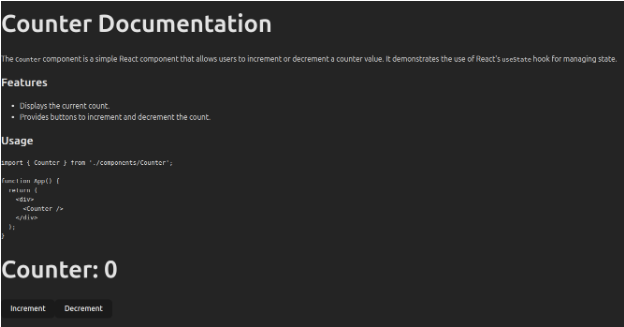
The counter component is rendered, and we can interact with it. That’s not all, we can also use JavaScript to create dynamic data, for example, to add a date to each document, we can now do:
# Counter Documentation
Date: {new Date().toLocaleDateString()}
And it will show:
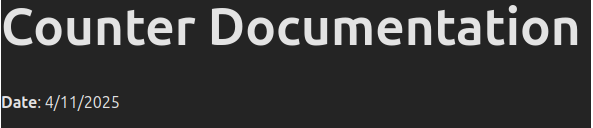
Let’s commit our new changes with git:
git add --all
git commit -m "Add readme and counter documents"
Finally, we can push our changes to a remote repository like GitHub.
git push origin main
We have created a new React project and integrated it with MDX in a couple of minutes!
Notes:
- MDX can be used with other popular web frameworks like Vue, Preact, Astro, NextJS, or in plain JavaScript or Node applications.
- You don’t necessarily need to render to HTML; you can transform your MDX files to other formats like PDF, Word, etc. This is done by adding plugins to the underlying ecosystem that supports MDX: https://github.com/remarkjs/remark, but that's a topic for another post.
- A commonly added syntax is GFM (GitHub Flavored Markdown), which can be enabled by adding the remark-gfm plugin, there is also support for math expressions using remark-math. And of course, you can also create your own plugins to enable and extend the syntax of MDX.
Summary
Docs as Code bridges the gap between development and documentation, fostering a culture of collaboration and agility. By adopting its practices, teams ensure their docs evolve alongside their software, reducing friction and boosting quality. Ready to start? Pick a tool, integrate docs into your next PR, and watch your documentation—and your product—thrive.